Build React Native App (4) - Redux, Jest, and NativeBase
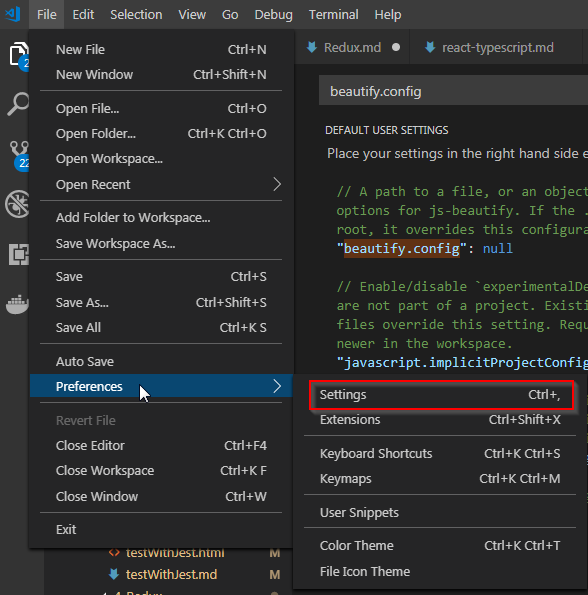
title: Replacing text in PDF file using iTextSharp
tags:
- PDF
- Replace Text
- iTextSharp
date: Nov 11, 2016
I’ve trying to replace text in PDF file and this is most simple way to replace text in PDF files. Before approaching this, I’ve tried to replace text using command toolkit with pdftk, qpdf to decrypt, and sfk181 to replace string with new, but this approach faced couple of issues; 1. font, 2. text area size.
After long investigation, this may be easy way to replace text. Put white colored rectangle on top of string and stamp text on the location like erase text using whitner and write on top of it.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using iTextSharp.text.pdf;
using iTextSharp.text.pdf.parser;
using iTextSharp.text;
using System.IO;
namespace EMTechnicalDieline
{
class Program
{
static void Main(string[] args)
{
string fileNameExisting = @"d:\temp\testpdf\TECH10031673.pdf";
string fileNameNew = @"d:\temp\testpdf\TECH10031673-new.pdf";
//variables
string pathin = fileNameExisting;
string pathout = fileNameNew;
using (PdfReader reader = new PdfReader(pathin))
using (PdfStamper stamper = new PdfStamper(reader, new FileStream(pathout, FileMode.Create)))
{
//select two pages from the original document
reader.SelectPages("1-1");
//gettins the page size in order to substract from the iTextSharp coordinates
var pageSize = reader.GetPageSize(1);
// PdfContentByte from stamper to add content to the pages over the original content
PdfContentByte pbover = stamper.GetOverContent(1);
//pbover.SaveState();
// for better coorniate, it would be better use pageSize object and calculate from top like text position in the below
iTextSharp.text.Rectangle rectangle = new iTextSharp.text.Rectangle(1200, 1850, 1530, 1890);
rectangle.BackgroundColor = BaseColor.WHITE;
pbover.Rectangle(rectangle);
BaseFont arialn = BaseFont.CreateFont("c:\\windows\\fonts\\Arialnb.ttf", BaseFont.CP1252, BaseFont.EMBEDDED);
Font font = new Font(arialn, 44);
//Font font = new Font();
// font.Size = 45;
//setting up the X and Y coordinates of the document
int x = 1300;
int y = 160;
x += 113;
y = (int)(pageSize.Height - y);
ColumnText.ShowTextAligned(pbover, Element.ALIGN_CENTER, new Phrase("12345678", font), x, y, 0);
}
}
}
}
There are so many ways to accomplish this like using form field, handling PDF format directly, replacing text only using command line tool etc, but I found out this is the best method if form field can’t be used.
I think this is a really good article. You make this information interesting and engaging. You give readers a lot to think about and I appreciate that kind of writing. view
ReplyDelete