Build React Native App (4) - Redux, Jest, and NativeBase
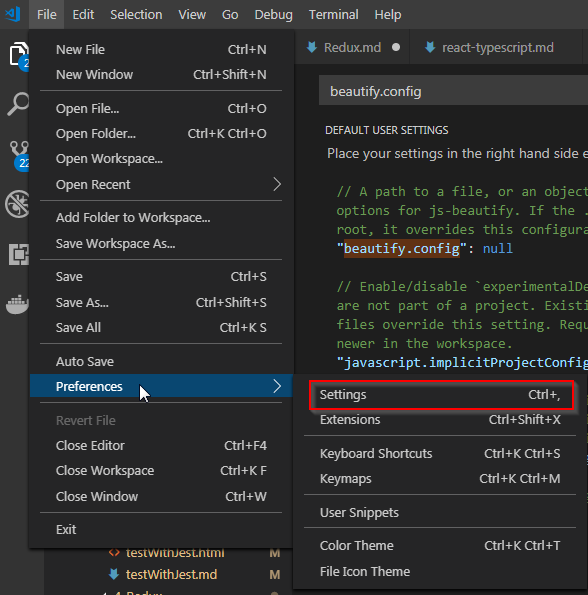
title: Developing Electron application with boilerpate, typescript, unit testing, bootstrap 4 and angularjs 2 (1) tags:
###Prequistics
###Reference
##Set up Angualr 2 and Electron Please refer homepage from bolerplate site. Skipping the details Modified clone command to change "electron-bolerplate" folder to "app"
git clone https://github.com/szwacz/electron-boilerplate.git project
# I deleted .\project\.git folder to include the source code into my git repo
cd project
npm install
npm start
I had an error, "node-pre-gyp can't find module internal/fs" when I used with node.js version 7.2.1. Please use version 6 for this boilerplate.
Bootstrap 4 uses scss format instead of less which is used in Bootstrap 3. To merge less, scss, and css files to one css file, following information will be modified
Filename: .\gulpfile.js
"dependencies": {
"fs-jetpack": "^0.10.2",
/* added code */
"bootstrap": "^4.0.0-alpha.5"
/* ----------- */
},
"devDependencies": {
"chai": "^3.5.0",
"electron": "^1.4.7",
"electron-builder": "^8.6.0",
"electron-mocha": "^3.0.0",
"gulp": "^3.9.0",
"gulp-batch": "^1.0.5",
"gulp-less": "^3.0.3",
/* added code */
"gulp-sass": "^2.0.4",
"gulp-concat": "^2.6.1",
"gulp-minify-css": "^1.2.1",
/* ----------- */
"gulp-plumber": "^1.1.0",
"gulp-util": "^3.0.6",
"gulp-watch": "^4.3.5",
"istanbul": "^0.4.3",
"merge-stream": "^1.0.0",
"minimist": "^1.2.0",
"mocha": "^3.0.2",
"rollup": "^0.36.3",
"rollup-plugin-istanbul": "^1.1.0",
"source-map-support": "^0.4.2",
"spectron": "^3.3.0"
}
Filename: .\tasks\build_app.js
......
var gulp = require('gulp');
var less = require('gulp-less');
/* added code */
var sass = require('gulp-sass');
var merge = require('merge-stream');
var concat = require('gulp-concat');
var minify = require('gulp-minify-css');
/* ----------- */
var watch = require('gulp-watch');
var batch = require('gulp-batch');
var plumber = require('gulp-plumber');
var jetpack = require('fs-jetpack');
var bundle = require('./bundle');
var utils = require('./utils');
gulp.task('less', function () {
......
gulp.task('less', function () {
var lessStream = gulp.src(srcDir.path('stylesheets/main.less'))
.pipe(plumber())
.pipe(less())
.pipe(concat('main.less'));
var scssStream = gulp.src(projectDir.path('node_modules/bootstrap/scss/*.scss'))
.pipe(plumber())
.pipe(sass())
.pipe(concat('bootstrap.scss'));
var cssStream = gulp.src(srcDir.path('stylesheets/main.css'))
.pipe(plumber())
.pipe(concat('styles.css'));
return merge(lessStream, scssStream, cssStream)
.pipe(concat('main.css'))
.pipe(minify())
.pipe(gulp.dest(destDir.path('stylesheets')));
});
......
gulp.task('watch', function () {
var beepOnError = function (done) {
return function (err) {
if (err) {
utils.beepSound();
}
done(err);
};
};
watch('src/**/*.js', batch(function (events, done) {
gulp.start('bundle', beepOnError(done));
}));
watch('src/**/*.less', batch(function (events, done) {
gulp.start('less', beepOnError(done));
}));
watch('node_modules/bootstrap/scss/**/*.scss', batch(function (events, done) {
gulp.start('less', beepOnError(done));
}));
});
filename: .\app\app.html
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>Electron Boilerplate</title>
<link href="stylesheets/main.css" rel="stylesheet" type="text/css">
<script src="helpers/context_menu.js"></script>
<script src="helpers/external_links.js"></script>
<script src="app.js"></script>
</head>
<body>
<div class="container">
<h1 id="greet"></h1>
<p class="subtitle">
Welcome to <a href="http://electron.atom.io" class="js-external-link">Electron</a> app running on this magnificent <strong id="platform-info"></strong> machine.
</p>
<p class="subtitle">
You are in <strong id="env-name"></strong> environment.
</p>
</div>
<div class="container">
<div class="row">
<div class="col-sm-4">.col-sm-4</div>
<div class="col-sm-4">.col-sm-4</div>
<div class="col-sm-4">.col-sm-4</div>
</div>
</div>
<!-- testing line for the bootstrap -->
<h3 class="text-success"> Bootstrap loaded</h3>
<!-- end testing line -->
</body>
</html>
To check whether all files are merged into one file, check .\app\stylesheets\main.css file
This is my first experience to build with electron with bootstrap and I really loves it. I am planning to build book reader with this platform and as I have progress, I will keep posting the progress.
Just wish to say your article is as astounding. The clearness in your
ReplyDeletesubmit is just excellent and i can suppose you’re knowledgeable in this subject.
Well along with your permission allow me to take hold of your RSS feed to stay up
to date with drawing close post. Thanks a million and
please carry on the rewarding work.
Read More: http://www.credosystemz.com/training-in-chennai/best-angularjs-training-in-chennai/