Build React Native App (4) - Redux, Jest, and NativeBase
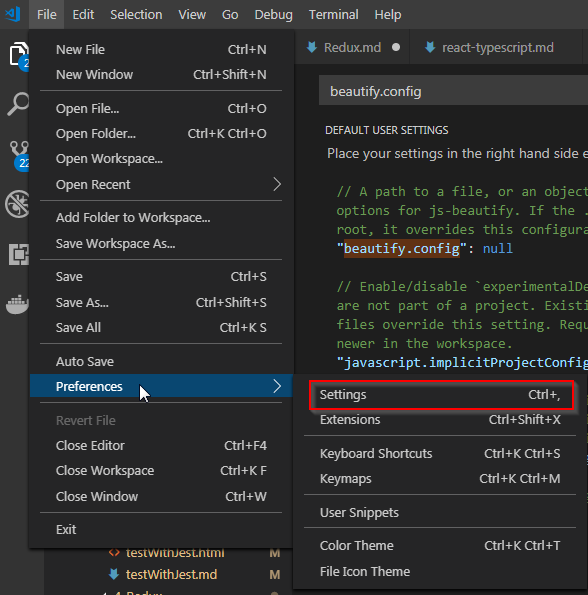
From this blog, the redux library will be added to handle REST service. There are so many good tutorial from blogs and Youtube about the Redux, so I am skipping the redux introduction.
D:\GitRepo\reactnative>npm --version
6.3.0
D:\GitRepo\reactnative>react-native --version
react-native-cli: 2.0.1
react-native: n/a - not inside a React Native project directory
D:\GitRepo\reactnative>yarn --version
1.9.4
$ react-native init ex3
If you want to specify the version, add "--version 0.57.3" at the end.
$ npm install native-base --save
...
+ native-base@2.8.1
added 71 packages from 42 contributors, removed 50 packages, updated 829 packages and audited 34989 packages in 138.542s
found 0 vulnerabilities
$
$ yarn
yarn install v1.9.4
warning package-lock.json found. Your project contains lock files generated by tools other than Yarn. It is advised not to mix package managers in order to avoid resolution inconsistencies caused by unsynchronized lock files. To clear this warning, remove package-lock.json.
[1/4] Resolving packages...
[2/4] Fetching packages...
...
[4/4] Building fresh packages...
success Saved lockfile.
Done in 25.75s.
$
$ react-native link
rnpm-install info Linking assets to ios project
rnpm-install WARN ERRGROUP Group 'Resources' does not exist in your Xcode project. We have created it automatically for you.
rnpm-install info Linking assets to android project
rnpm-install info Assets have been successfully linked to your project
I am big fan to move the terminal from bottom to the right side and split it to two; one is for jest and the other is to run app.
TDD(Test Driven Development) is almost mandatory knowledge for any developers.
Testing has two types of testing. One is unit testing and the other is integration testing. The unit testing is for testing to check the function or module itself(In other words, testing only one thing) and the integration testing is for testing multiple things such as some logic among modules or functions.
The recommendation to accomodate these two difference cases, the unit testing stores at the same place as source code and the integration testing stores at the different folder.
src/
├─module1
│ ├─index.js
│ ├─add.js
│ └─add.unit.test.js
├─module2
│ ├─index.js
│ ├─subtract.js
│ └─subtract.unit.test.js
└─index.js
tests
├─integration1
│ └─api.int.test.js (int stands for integration)
└─components
└─restComponent.int.test.js
add add.js file
export const add = (arg1, arg2) => arg1 + arg2;
add add.unit.test.js file
import {add} from './add';
test('add', () => {
expect(add(1,2)).toBe(3);
})
Result
PASS src/redux/actions/yahooRestAction.test.js
√ yahooRestAction (4ms)
PASS src/module1/add.unit.test.js
√ add (4ms)
Test Suites: 2 passed, 2 total
Tests: 2 passed, 2 total
Snapshots: 0 total
Time: 2.148s
Ran all test suites.
Watch Usage
› Press f to run only failed tests.
› Press o to only run tests related to changed files.
› Press p to filter by a filename regex pattern.
› Press t to filter by a test name regex pattern.
› Press q to quit watch mode.
› Press Enter to trigger a test run.
Run following command
$ yarn add metro-react-native-babel-preset --dev
...
+ metro-react-native-babel-preset@0.48.1
added 3 packages from 1 contributor, updated 1 package and
audited 35018 packages in 10.951s
found 0 vulnerabilities
$ yarn
...
$
Update package.json file
{
"name": "ex3",
"version": "0.0.1",
"private": true,
"scripts": {
"start": "node node_modules/react-native/local-cli/cli.js start",
"test": "jest --verbose",
"test-watch": "jest --watchAll --verbose"
},
"dependencies": {
"enzyme": "^3.7.0",
"native-base": "^2.8.1",
"react": "16.6.0-alpha.8af6728",
"react-native": "0.57.3",
"react-redux": "^5.0.7"
},
"devDependencies": {
"babel-jest": "23.6.0",
"jest": "23.6.0",
"metro-react-native-babel-preset": "^0.48.1",
"react-test-renderer": "16.6.0-alpha.8af6728"
},
"jest": {
"preset": "react-native",
"moduleFileExtensions": [
"ts",
"tsx",
"js"
],
"transform": {
"^.+\\.js$": "<rootDir>/node_modules/react-native/jest/preprocessor.js"
},
"testRegex": "(/__tests__/.*|\\.(test|spec))\\.(ts|tsx|js)$",
"testPathIgnorePatterns": [
"\\.snap$",
"<rootDir>/node_modules/"
],
"cacheDirectory": ".jest/cache"
}
}
Add following lines to the .gitignore file.
...
# JEST
\.jest/
Full list in .gitignore file
# OSX
#
.DS_Store
# Xcode
#
build/
*.pbxuser
!default.pbxuser
*.mode1v3
!default.mode1v3
*.mode2v3
!default.mode2v3
*.perspectivev3
!default.perspectivev3
xcuserdata
*.xccheckout
*.moved-aside
DerivedData
*.hmap
*.ipa
*.xcuserstate
project.xcworkspace
# Android/IntelliJ
#
build/
.idea
.gradle
local.properties
*.iml
# node.js
#
node_modules/
npm-debug.log
yarn-error.log
# BUCK
buck-out/
\.buckd/
*.keystore
# fastlane
#
# It is recommended to not store the screenshots in the git repo. Instead, use fastlane to re-generate the
# screenshots whenever they are needed.
# For more information about the recommended setup visit:
# https://docs.fastlane.tools/best-practices/source-control/
*/fastlane/report.xml
*/fastlane/Preview.html
*/fastlane/screenshots
# Bundle artifact
*.jsbundle
# JEST
\.jest/
From next tutorial, we will add redux components with unit testing.
DOPBNK
ReplyDelete