Build React Native App (4) - Redux, Jest, and NativeBase
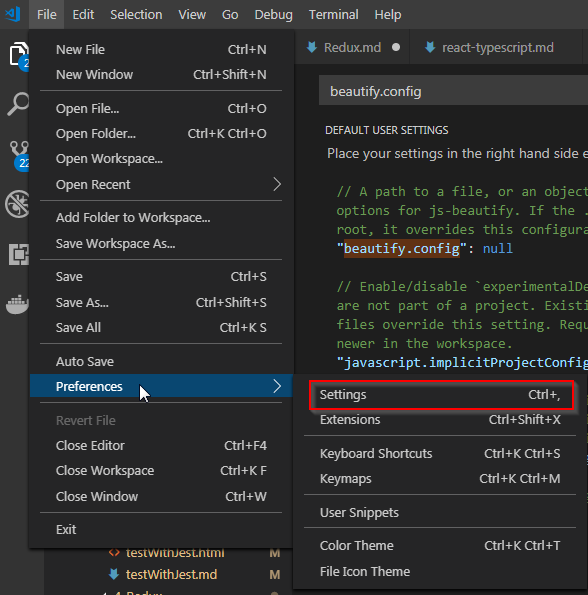
title: Build Spring Boot and Angular Example(1) - Set up environment tags:
Lots of article explained how to build AngularJS or Spring Boot separately, but I couldn't find any good article how I can package these two components together and running from one package. The best way to do this is to separate back-end and front-end using REST api and packaging as one. Spring Boot has built-in components, so both front-end and back-end will be running from the Spring Boot Web components.
For the Spring Boot app(Back End), STS IDE will be used.
For the AngualarJS 2(Front End), following technologies will be used;
There is another way to develop UI using AngularJS material, but I am going to use Bootstrap as my UI library.
To do this, we will need two routing URLs, one is for AnguarlJS and the other is for SpringBoot REST.
From this example, we will build CRUD operation for item and account.
The Routing Path for the angularJS will be
The Routing path for the SpringBoot REST will be
First of all, NodeJS should be updated with latest version, so please go to NodeJS and install latest versoin
D:\test\angulartest>node -v
v8.4.0
D:\test\angulartest>npm -v
5.3.0
D:\test\angulartest>
After installing NodeJS, angular cli should be installed to use boilerplate and generate source code using ngcommand
ng new: The Angular CLI makes it easy to create an application that already works, right out of the box. It already follows our best practices!
ng generate: Generate components, routes, services and pipes with a simple command. The CLI will also create simple test shells for all of these.
ng serve: Easily test your app locally while developing.
Test, Lint, Format: Make your code really shine. Run your unittests or your end-to-end tests with the breeze of a command. Execute the official Angular linter and run clang format.
This components should be install to global repository.(option -g)
D:\test\angulartest>npm install -g @angular/cli
C:\Users\alex.joh\AppData\Roaming\npm\ng -> C:\Users\alex.joh\AppData\Roaming\npm\node_modules\@angu
lar\cli\bin\ng
npm WARN optional SKIPPING OPTIONAL DEPENDENCY: fsevents@1.1.2 (node_modules\@angular\cli\node_modul
es\fsevents):
npm WARN notsup SKIPPING OPTIONAL DEPENDENCY: Unsupported platform for fsevents@1.1.2: wanted {"os":
"darwin","arch":"any"} (current: {"os":"win32","arch":"x64"})
+ @angular/cli@1.4.0
added 156 packages, removed 77 packages, updated 27 packages and moved 2 packages in 61.281s
D:\test\angulartest>
D:\test\angulartest>ng -v
Your global Angular CLI version (1.4.0) is greater than your local
version (1.3.2). The local Angular CLI version is used.
To disable this warning use "ng set --global warnings.versionMismatch=false".
_ _ ____ _ ___
/ \ _ __ __ _ _ _| | __ _ _ __ / ___| | |_ _|
/ △ \ | '_ \ / _` | | | | |/ _` | '__| | | | | | |
/ ___ \| | | | (_| | |_| | | (_| | | | |___| |___ | |
/_/ \_\_| |_|\__, |\__,_|_|\__,_|_| \____|_____|___|
|___/
@angular/cli: 1.3.2
node: 8.4.0
os: win32 x64
@angular/animations: 4.3.6
@angular/common: 4.3.6
@angular/compiler: 4.3.6
@angular/core: 4.3.6
@angular/forms: 4.3.6
@angular/http: 4.3.6
@angular/platform-browser: 4.3.6
@angular/platform-browser-dynamic: 4.3.6
@angular/router: 4.3.6
@angular/cli: 1.3.2
@angular/compiler-cli: 4.3.6
@angular/language-service: 4.3.6
D:\test\angulartest>
D:\test>ng new testapp
create testapp/e2e/app.e2e-spec.ts (289 bytes)
create testapp/e2e/app.po.ts (208 bytes)
create testapp/e2e/tsconfig.e2e.json (235 bytes)
create testapp/karma.conf.js (923 bytes)
create testapp/package.json (1312 bytes)
create testapp/protractor.conf.js (722 bytes)
create testapp/README.md (1098 bytes)
create testapp/tsconfig.json (363 bytes)
create testapp/tslint.json (3040 bytes)
create testapp/.angular-cli.json (1125 bytes)
create testapp/.editorconfig (245 bytes)
create testapp/.gitignore (516 bytes)
create testapp/src/assets/.gitkeep (0 bytes)
create testapp/src/environments/environment.prod.ts (51 bytes)
create testapp/src/environments/environment.ts (387 bytes)
create testapp/src/favicon.ico (5430 bytes)
create testapp/src/index.html (294 bytes)
create testapp/src/main.ts (370 bytes)
create testapp/src/polyfills.ts (2480 bytes)
create testapp/src/styles.css (80 bytes)
create testapp/src/test.ts (1085 bytes)
create testapp/src/tsconfig.app.json (211 bytes)
create testapp/src/tsconfig.spec.json (304 bytes)
create testapp/src/typings.d.ts (104 bytes)
create testapp/src/app/app.module.ts (314 bytes)
create testapp/src/app/app.component.html (1075 bytes)
create testapp/src/app/app.component.spec.ts (986 bytes)
create testapp/src/app/app.component.ts (207 bytes)
create testapp/src/app/app.component.css (0 bytes)
Installing packages for tooling via npm.
Installed packages for tooling via npm.
Project 'testapp' successfully created.
D:\test>cd testapp
D:\test\testapp>npm install --save bootstrap@4.0.0-alpha.6 font-awesome
npm WARN optional SKIPPING OPTIONAL DEPENDENCY: fsevents@1.1.2 (node_modules\fsevents):
npm WARN notsup SKIPPING OPTIONAL DEPENDENCY: Unsupported platform for fsevents@1.1.2: wanted {"os":
"darwin","arch":"any"} (current: {"os":"win32","arch":"x64"})
+ bootstrap@4.0.0-alpha.6
+ font-awesome@4.7.0
added 118 packages in 17.369s
D:\test\testapp>ng serve
Port 4200 is already in use. Use '--port' to specify a different port.
D:\test\testapp>ng serve
** NG Live Development Server is listening on localhost:4200, open your browser on http://localhost:
4200/ **
Date: 2017-09-07T19:20:35.387Z
Hash: f0ada5e206130a902126
Time: 5707ms
chunk {inline} inline.bundle.js, inline.bundle.js.map (inline) 5.83 kB [entry] [rendered]
chunk {main} main.bundle.js, main.bundle.js.map (main) 8.64 kB {vendor} [initial] [rendered]
chunk {polyfills} polyfills.bundle.js, polyfills.bundle.js.map (polyfills) 209 kB {inline} [initial]
[rendered]
chunk {styles} styles.bundle.js, styles.bundle.js.map (styles) 11.3 kB {inline} [initial] [rendered]
chunk {vendor} vendor.bundle.js, vendor.bundle.js.map (vendor) 2.28 MB [initial] [rendered]
webpack: Compiled successfully.
After running application
Adding bootstrap
From TestApp.angular-cli.json, add bootstrap.min.css under "styles"
"testTsconfig": "tsconfig.spec.json",
"prefix": "app",
"styles": [
"styles.css",
"../node_modules/bootstrap/dist/css/bootstrap.min.css"
],
"scripts": [],
"environmentSource": "environments/environment.ts",
To test Bootstrap, "jumbotron" is added to the top of html.
filename: TestApp\src\app\app.component.html
<!--The content below is only a placeholder and can be replaced.-->
<div class="container">
<div class="jumbotron">
<h1 class="display-3">Bootstrap Test</h1>
</div>
</div>
<div style="text-align:center">
<h1>
Welcome to {{title}}!
</h1>
Result after applying bootstrap
If you are running this from IE, there will be error like; File: polyfills.bundle.js, Line: 859, Column: 36
To fix this, go to polyfills.ts file and uncomment all lines related IE and run npm command as instructed. Need to install two more files.
/** IE9, IE10 and IE11 requires all of the following polyfills. **/
import 'core-js/es6/symbol';
import 'core-js/es6/object';
import 'core-js/es6/function';
import 'core-js/es6/parse-int';
import 'core-js/es6/parse-float';
import 'core-js/es6/number';
import 'core-js/es6/math';
import 'core-js/es6/string';
import 'core-js/es6/date';
import 'core-js/es6/array';
import 'core-js/es6/regexp';
import 'core-js/es6/map';
import 'core-js/es6/weak-map';
import 'core-js/es6/set';
/** IE10 and IE11 requires the following for NgClass support on SVG elements */
import 'classlist.js'; // Run `npm install --save classlist.js`.
/** Evergreen browsers require these. **/
import 'core-js/es6/reflect';
import 'core-js/es7/reflect';
/**
* Required to support Web Animations `@angular/animation`.
* Needed for: All but Chrome, Firefox and Opera. http://caniuse.com/#feat=web-animation
**/
import 'web-animations-js'; // Run `npm install --save web-animations-js`.
D:\test\testapp>npm install --save classlist.js
npm WARN optional SKIPPING OPTIONAL DEPENDENCY: fsevents@1.1.2 (node_modules\fsevents):
npm WARN notsup SKIPPING OPTIONAL DEPENDENCY: Unsupported platform for fsevents@1.1.2: wanted {"os":"darwin","arch":"any"} (current:
{"os":"win32","arch":"x64"})
+ classlist.js@1.1.20150312
added 115 packages in 20.059s
D:\test\testapp>npm install --save web-animations-js
npm WARN optional SKIPPING OPTIONAL DEPENDENCY: fsevents@1.1.2 (node_modules\fsevents):
npm WARN notsup SKIPPING OPTIONAL DEPENDENCY: Unsupported platform for fsevents@1.1.2: wanted {"os":"darwin","arch":"any"} (current:
{"os":"win32","arch":"x64"})
+ web-animations-js@2.3.1
added 115 packages in 10.477s
Run cmd as administrator Run npm config edit (You will get notepad editor) Change Prefix variable to C:\Users<User Name>\AppData\Roaming\npm
From this template, we accomplished how to set up AngularJS development and how to applying Bootstrap to angularjs.
Next tutorial will show how to create Spring Boot REST app and how to test with Postman
Thanks for sharing this. It is really helpful.
ReplyDeleteAngularJS Training |
AngularJS Training in Chennai | AngularJS course in Chennai